In this HackerRank Day 2 : Operators 30 days of code solution ,in this problem.
Task
Given the meal price (base cost of a meal), tip percent (the percentage of the meal price being added as tip), and tax percent (the percentage of the meal price being added as tax) for a meal, find and print the meal’s total cost. Round the result to the nearest integer.
Example
mealcost = 100
tip percent = 15
tax percent = 8
A tip of 15% * 100 = 15, and the taxes are 8% * 100 = 8. Print the value 123 and return from the function.
Function Description
Complete the solve function in the editor below.
solve has the following parameters:
- int meal_cost: the cost of food before tip and tax
- int tip_percent: the tip percentage
- int tax_percent: the tax percentage
Returns The function returns nothing. Print the calculated value, rounded to the nearest integer.
Note: Be sure to use precise values for your calculations, or you may end up with an incorrectly rounded result.
Input Format
There are lines of numeric input:
The first line has a double, mealcost (the cost of the meal before tax and tip).
The second line has an integer, tippercent (the percentage of being added as tip).
The third line has an integer, taxpercent(the percentage of being added as tax).
Sample Input
12.00 20 8
Sample Output
15
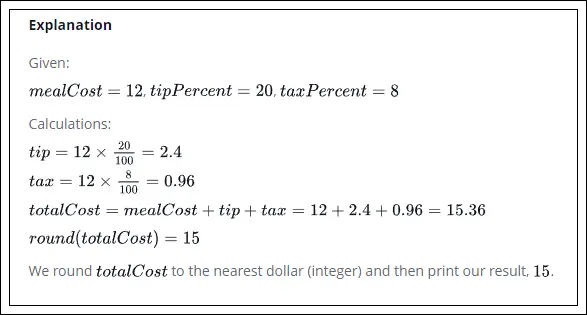
HackerRank Day 2 : Operators 30 days of code solution
Operators HackerRank Solution in C
#include <stdio.h> #include <string.h> #include <math.h> #include <stdlib.h> int main() { /* Enter your code here. Read input from STDIN. Print output to STDOUT */ float mealValue; int tip; int tax; unsigned int total; /* Enter your code here. Read input from STDIN. Print output to STDOUT */ scanf("%f",(float *)&mealValue); scanf("%d",&tip); scanf("%d",&tax); total = mealValue + (mealValue*((float)tip/100)) + (mealValue*((float)tax/100)) + .5; printf("The total meal cost is %d dollars.", (int)total); return 0; }
Operators HackerRank Solution in C ++
#include <cmath> #include <cstdio> #include <vector> #include <iostream> #include <algorithm> using namespace std; int main() { /* Enter your code here. Read input from STDIN. Print output to STDOUT */ double mealCost; double totalCost; int totalCostRound; int tipPercent; int taxPercent; cin >> mealCost >> tipPercent >> taxPercent; totalCost = mealCost * (1.0 + (double)taxPercent/100.0 + (double)tipPercent/100.0); totalCostRound = (int)(totalCost + 0.5); // cout << mealCost << ", " << tipPercent << ", " << taxPercent << endl; cout << "The total meal cost is " << totalCostRound << " dollars."; return 0; }
Operators HackerRank Solution in Java
import java.io.*; import java.util.*; import java.text.*; import java.math.*; import java.util.regex.*; public class Arithmetic { public static void main(String[] args) { Scanner scan = new Scanner(System.in); double mealCost = scan.nextDouble(); // original meal price int tipPercent = scan.nextInt(); // tip percentage int taxPercent = scan.nextInt(); // tax percentage scan.close(); double tip = mealCost * tipPercent / 100; double tax = mealCost * taxPercent / 100; double total = mealCost + tip + tax; // cast the result of the rounding operation to an int and save it as totalCost int totalCost = (int) Math.round(total); // Print your result System.out.println("The total meal cost is " + totalCost + " dollars."); } }
Operators HackerRank Solution in Python 3
#!/bin/python3 import math import os import random import re import sys # Complete the solve function below. def solve(meal_cost, tip_percent, tax_percent): tip = meal_cost * tip_percent / 100 tax = meal_cost * tax_percent / 100 total_cost = meal_cost + tip + tax print(round(total_cost)) if __name__ == '__main__': meal_cost = float(input()) tip_percent = int(input()) tax_percent = int(input()) solve(meal_cost, tip_percent, tax_percent)
Operators HackerRank Solution in JavaScript
function processData(input) { //Enter your code here var pieces = input.split('\n'); var mealCost = parseFloat(pieces[0]); var tipPercent = parseInt(pieces[1]); var taxPercent = parseInt(pieces[2]); var total = mealCost * tipPercent / 100 + mealCost * taxPercent / 100 + mealCost; console.log('The total meal cost is ' + Math.round(total) + ' dollars.') } process.stdin.resume(); process.stdin.setEncoding("ascii"); _input = ""; process.stdin.on("data", function (input) { _input += input; }); process.stdin.on("end", function () { processData(_input); });
NEXT : Hackerrank Day 3 : Intro to Conditional Statements
30 Days of Code HackerRank Solutions List – Day 0 to Day 29
Read More –
- How To Create Responsive Image Gallery Using Html & Css
- Create a Quiz App using HTML CSS & JavaScript
- Product Landing Page Using Html Css Bootstrap